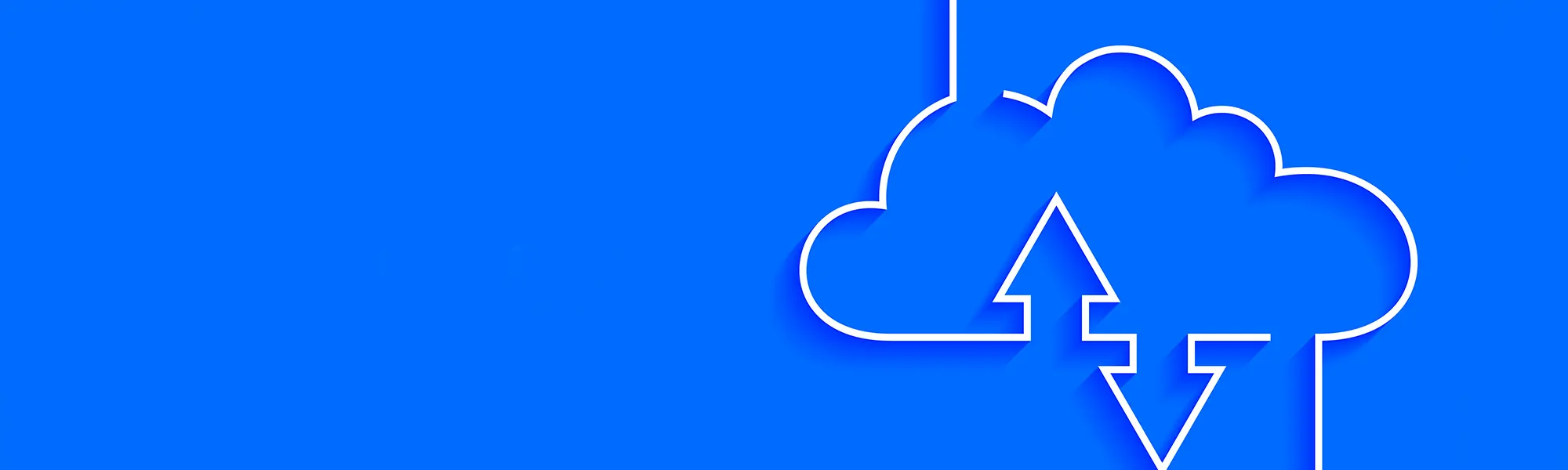
PHP Functions
Utilize these pre-developed functions to quickly build and enhance your integrations
Utilize these pre-developed functions to quickly build and enhance your integrations
Welcome to the official documentation of the Micra Max API PHP Functions, provided by Diseños y Tecnología S.A. (DITEL). This document aims to guide you through using our PHP functions to integrate seamlessly with the Micra Max device using its REST API.
API Overview
Our API allows you to communicate with the Micra Max device to perform a range of operations, from fetching current configurations to performing factory resets. These functions require the use of a valid API token (DT_PANEL_TOKEN) for authentication.
Configuration
Before you begin, please ensure that the API token is correctly defined in your PHP script. To do this, modify or edit the functions.php
file using a text editor. For example, we are demonstrating this process using the nano
text editor on Linux:
$ nano functions.php
functions.php
//API PHP Functions Ver 1.0 (06/10/2023)
//Diseños y tecnología S.A. (DITEL)
//https://www.ditel.es
//API_PASSWORD ES NECESARIO.
define("DT_PANEL_TOKEN", "your_api_token_here");
Replace your_api_token_here with the actual API token that webserver of MICRA MAX is having on configuration.
It's essential to have the cURL library installed on your system for the REST API library to function correctly. Additionally, it's very important to remember to include functions.php in your project.
PHP Functions
function micra_max_hello($ip){
$headers = array();
$headers[] = "X-DTpanel: ". DT_PANEL_TOKEN;
$headers[] = 'Content-type: application/json';
$state_ch = curl_init();
curl_setopt($state_ch, CURLOPT_URL,$ip."/v1/hello.json");
curl_setopt($state_ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($state_ch, CURLOPT_CUSTOMREQUEST, "GET");
curl_setopt($state_ch, CURLOPT_HTTPHEADER, $headers);
$response = curl_exec ($state_ch);
$result = json_decode($response, true);
return $result;
}
Description:
This function is very useful for verifying if the installation and installation parameters are correct. It is a simple function that will respond if everything is OK. It is very useful to test this function before performing more complex operations to ensure that the entire programming environment is set up correctly.
Parameters:
$ip
The IP address of the Micra Max device.
Returns: A JSON object containing the response.
function micra_max_get_config($ip){
$headers = array();
$headers[] = "X-DTpanel: ". DT_PANEL_TOKEN;
$headers[] = 'Content-type: application/json';
$state_ch = curl_init();
curl_setopt($state_ch, CURLOPT_URL,$ip."/v1/config");
curl_setopt($state_ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($state_ch, CURLOPT_CUSTOMREQUEST, "GET");
curl_setopt($state_ch, CURLOPT_HTTPHEADER, $headers);
$response = curl_exec ($state_ch);
$result = json_decode($response, true);
return $result;
}
Description: Retrieves the current configuration from the Micra Max device.
Parameters:
$ip
The IP address of the device.
Returns: A JSON object containing the current configuration.
function micra_max_get_display($ip){
$headers = array();
$headers[] = "X-DTpanel: ". DT_PANEL_TOKEN;
$headers[] = 'Content-type: application/json';
$state_ch = curl_init();
curl_setopt($state_ch, CURLOPT_URL,$ip."/v1/get_display");
curl_setopt($state_ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($state_ch, CURLOPT_CUSTOMREQUEST, "GET");
curl_setopt($state_ch, CURLOPT_HTTPHEADER, $headers);
$response = curl_exec ($state_ch);
$result = json_decode($response, true);
return $result;
}
Description: Fetches the current display settings of the Micra Max device.
Parameters:
$ip
The IP address of the device.
Returns: A JSON object with the display value.
function micra_max_get_info($ip){
$headers = array();
$headers[] = "X-DTpanel: ". DT_PANEL_TOKEN;
$headers[] = 'Content-type: application/json';
$state_ch = curl_init();
curl_setopt($state_ch, CURLOPT_URL,$ip."/v1/get_info");
curl_setopt($state_ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($state_ch, CURLOPT_CUSTOMREQUEST, "GET");
curl_setopt($state_ch, CURLOPT_HTTPHEADER, $headers);
$response = curl_exec ($state_ch);
$result = json_decode($response, true);
return $result;
}
Description: Obtains detailed information about the Micra Max device.
Parameters:
$ip
The IP address of the device.
Returns: A JSON object with detailed information.
function micra_max_reset_tare($ip){
$headers = array();
$headers[] = "X-DTpanel: ". DT_PANEL_TOKEN;
$headers[] = 'Content-type: application/json';
$state_ch = curl_init();
curl_setopt($state_ch, CURLOPT_URL,$ip."/v1/reset_tare");
curl_setopt($state_ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($state_ch, CURLOPT_CUSTOMREQUEST, "POST");
curl_setopt($state_ch, CURLOPT_HTTPHEADER, $headers);
$response = curl_exec ($state_ch);
$result = json_decode($response, true);
return $result;
}
Description: Resets the tare weight on the Micra Max device.
Parameters:
$ip
The IP address of the device.
Returns: A JSON object confirming the reset.
function micra_max_reset_max($ip){
$headers = array();
$headers[] = "X-DTpanel: ". DT_PANEL_TOKEN;
$headers[] = 'Content-type: application/json';
$state_ch = curl_init();
curl_setopt($state_ch, CURLOPT_URL,$ip."/v1/reset_max");
curl_setopt($state_ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($state_ch, CURLOPT_CUSTOMREQUEST, "POST");
curl_setopt($state_ch, CURLOPT_HTTPHEADER, $headers);
$response = curl_exec ($state_ch);
$result = json_decode($response, true);
return $result;
}
function micra_max_reset_min($ip){
$headers = array();
$headers[] = "X-DTpanel: ". DT_PANEL_TOKEN;
$headers[] = 'Content-type: application/json';
$state_ch = curl_init();
curl_setopt($state_ch, CURLOPT_URL,$ip."/v1/reset_min");
curl_setopt($state_ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($state_ch, CURLOPT_CUSTOMREQUEST, "POST");
curl_setopt($state_ch, CURLOPT_HTTPHEADER, $headers);
$response = curl_exec ($state_ch);
$result = json_decode($response, true);
return $result;
}
Description: Resets the maximum/minimum recorded values.
Parameters:
$ip
The IP address of the device.
Returns: A JSON object confirming the reset.
function micra_max_tare($ip){
$headers = array();
$headers[] = "X-DTpanel: ". DT_PANEL_TOKEN;
$headers[] = 'Content-type: application/json';
$state_ch = curl_init();
curl_setopt($state_ch, CURLOPT_URL,$ip."/v1/tare");
curl_setopt($state_ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($state_ch, CURLOPT_CUSTOMREQUEST, "POST");
curl_setopt($state_ch, CURLOPT_HTTPHEADER, $headers);
$response = curl_exec ($state_ch);
$result = json_decode($response, true);
return $result;
}
Description: Sets the current weight as the tare weight.
Parameters:
$ip
The IP address of the device.
Returns: A JSON object confirming the operation.
function micra_max_factory_reset($ip){
$headers = array();
$headers[] = "X-DTpanel: ". DT_PANEL_TOKEN;
$headers[] = 'Content-type: application/json';
$state_ch = curl_init();
curl_setopt($state_ch, CURLOPT_URL,$ip."/v1/fatory_reset");
curl_setopt($state_ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($state_ch, CURLOPT_CUSTOMREQUEST, "POST");
curl_setopt($state_ch, CURLOPT_HTTPHEADER, $headers);
$response = curl_exec ($state_ch);
$result = json_decode($response, true);
return $result;
}
Description: Performs a factory reset on the Micra Max device, restoring default settings.
Parameters:
$ip
The IP address of the device.
Returns: A JSON object confirming the reset.
function micra_max_post_config($ip,$array){
$api_data_json = json_encode($array);
$headers = array();
$headers[] = "X-DTpanel: ". DT_PANEL_TOKEN;
$headers[] = 'Content-type: application/json';
$state_ch = curl_init();
curl_setopt($state_ch, CURLOPT_URL,$ip."/v1/config");
curl_setopt($state_ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($state_ch, CURLOPT_CUSTOMREQUEST, "POST");
curl_setopt($state_ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($state_ch, CURLOPT_POSTFIELDS, $api_data_json);
$response = curl_exec ($state_ch);
$result = json_decode($response, true);
}
Description: Posts a new configuration to the Micra Max device.
Parameters:
$ip
The IP address of the device.
$array: An associative array containing the new configuration settings.
Returns: A JSON object confirming the update.
Example Usage
example.php
include_once('functions.php') //We include the library
$ip = "192.168.1.1"; // Replace with your device's IP address
$result = micra_max_hello($ip); // We call to the function with the $ip parameter
print_r($result); //We print the result
Another Example: This example will display on a webpage the display value each second
//*****************************
//index.php (Java script code)
//*****************************
function actualizarValor() {
// Realizar una solicitud AJAX para obtener el valor actualizado
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
// Actualizar el valor en la página
document.getElementById("resultado").innerHTML = this.responseText;
}
};
xhttp.open("GET", "consulta_valor.php", true);
xhttp.send();
}
// Actualizar el valor cada segundo
setInterval(actualizarValor, 1000);
//*********************************
//consulta_valor.php (php script)
//*********************************
require_once('functions.php');
$ip = "10.0.0.226";
$result = micra_max_get_display($ip);
echo $result["bigDisplayValue"];
For further assistance or to report issues, please contact our support team at this contact form