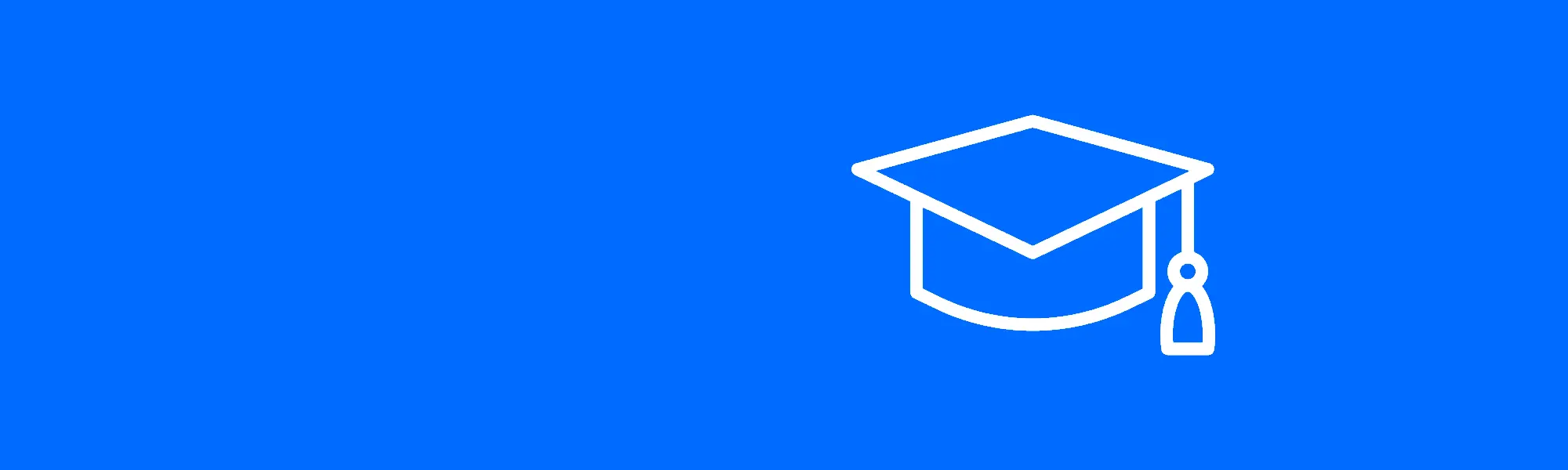
TUTORIALS
Learn all about MICRA MAX
Latest blog posts
How to display the value from a Micra Max on a PC monitor via a Web Browser
Fecha: 2024-08-28 09:59:12Autor: Alex Rubio
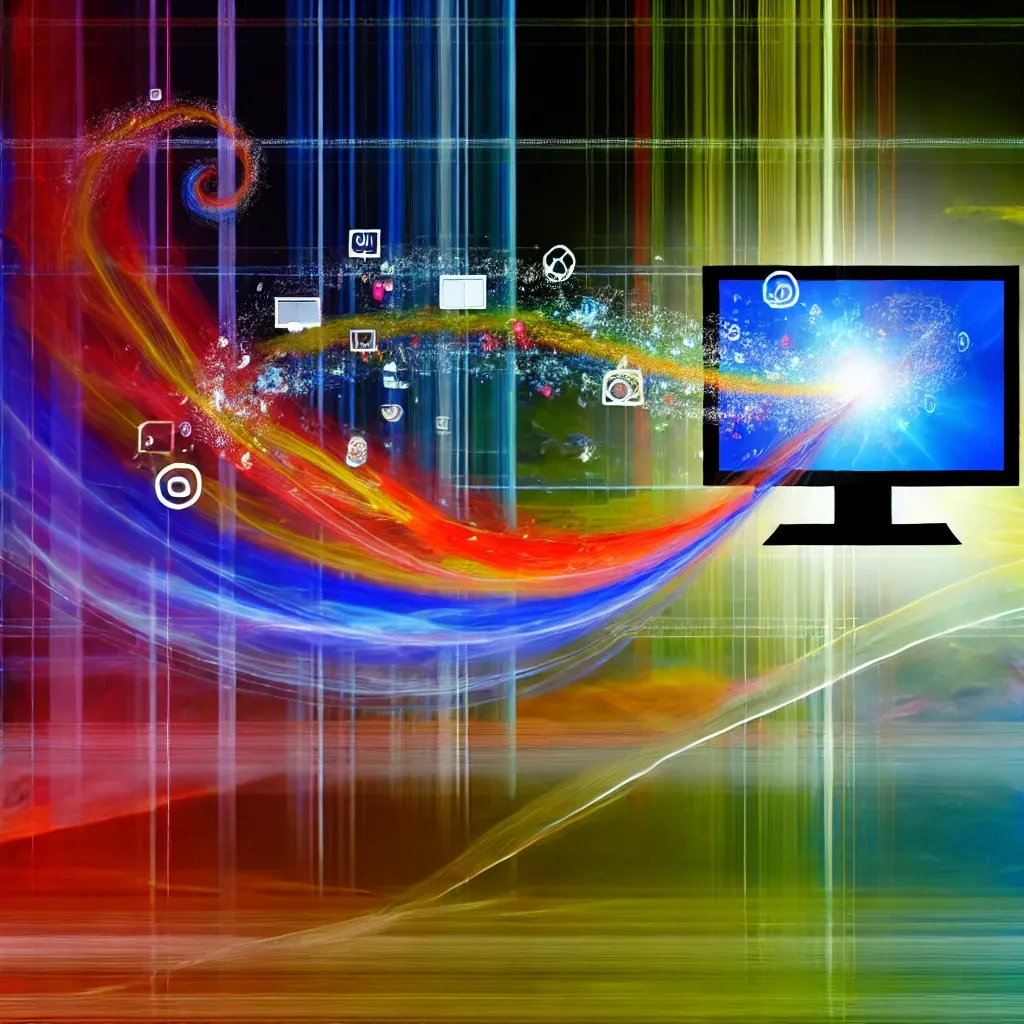
Step-by-Step Guide
Follow these steps to display the value from a Micra Max device on a PC monitor through a web browser using JavaScript and PHP.
1. Set Up the Web Server
To begin, ensure you have a web server set up to host your PHP files. You can use software like XAMPP or WAMP for a local environment.
2. Create the JavaScript File to Update the Display Value
You will need a JavaScript file to periodically request the value from the Micra Max device and display it on a webpage. Create an index.php
file in the root directory of your web server with the following content:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Micra Max Display Value</title>
<script>
function updateValue() {
// Create an AJAX request to fetch the updated value
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
// Update the value on the page
document.getElementById("result").innerHTML = this.responseText;
}
};
xhttp.open("GET", "get_value.php", true);
xhttp.send();
}
// Update the value every second
setInterval(updateValue, 1000);
</script>
</head>
<body>
<h1>Micra Max Display Value</h1>
<div id="result">Loading...</div>
</body>
</html>
Explanation: This JavaScript function, updateValue()
, uses AJAX to call a PHP script (get_value.php
) every second. The result of the call is then displayed in the div
with the id result
.
3. Create the PHP Script to Fetch the Value
Next, create a PHP script named get_value.php
. This script will connect to the Micra Max device and fetch the display value.
<?php
// Load the required functions
require_once('functions.php');
// Specify the IP address of the Micra Max device
$ip = "10.0.0.226";
$token = "XXXXXXXXXXXXX";
// Fetch the display value from the Micra Max device
$result = micra_max_get_display($ip,$token);
// Output the fetched value
echo $result["bigDisplayValue"];
?>
Explanation: This PHP script uses the function micra_max_get_display()
(assumed to be defined in functions.php
) to retrieve the display value from the Micra Max device at the specified IP address (10.0.0.226
). The result is then returned to the JavaScript code.
4. Download the Functions File
Make sure you have a file named functions.php
that contains the necessary function to communicate with the Micra Max device.
You can download it from GitHub
Explanation: The micra_max_get_display()
function should contain the actual logic to connect to the Micra Max device and retrieve its display value. The provided example shows a mock response structure.
5. Run the Web Server and Open the Webpage
- Start your web server (e.g., Apache in XAMPP or WAMP).
- Place all the files (
index.php
,get_value.php
,functions.php
) in the appropriate directory (usually thehtdocs
orwww
folder of your web server). - Open your web browser and navigate to
http://localhost/index.php
.
6. Verify the Display
Once you open the webpage, you should see the display value from the Micra Max device being updated every second.
Conclusion
By following these steps, you can continuously display the value from a Micra Max device on a webpage using JavaScript and PHP. This allows you to monitor the device's display in real-time from any PC with a web browser connected to your local network.
What is an Endpoint in a REST API?
Fecha: 2024-08-28 09:36:57Autor: Alex Rubio
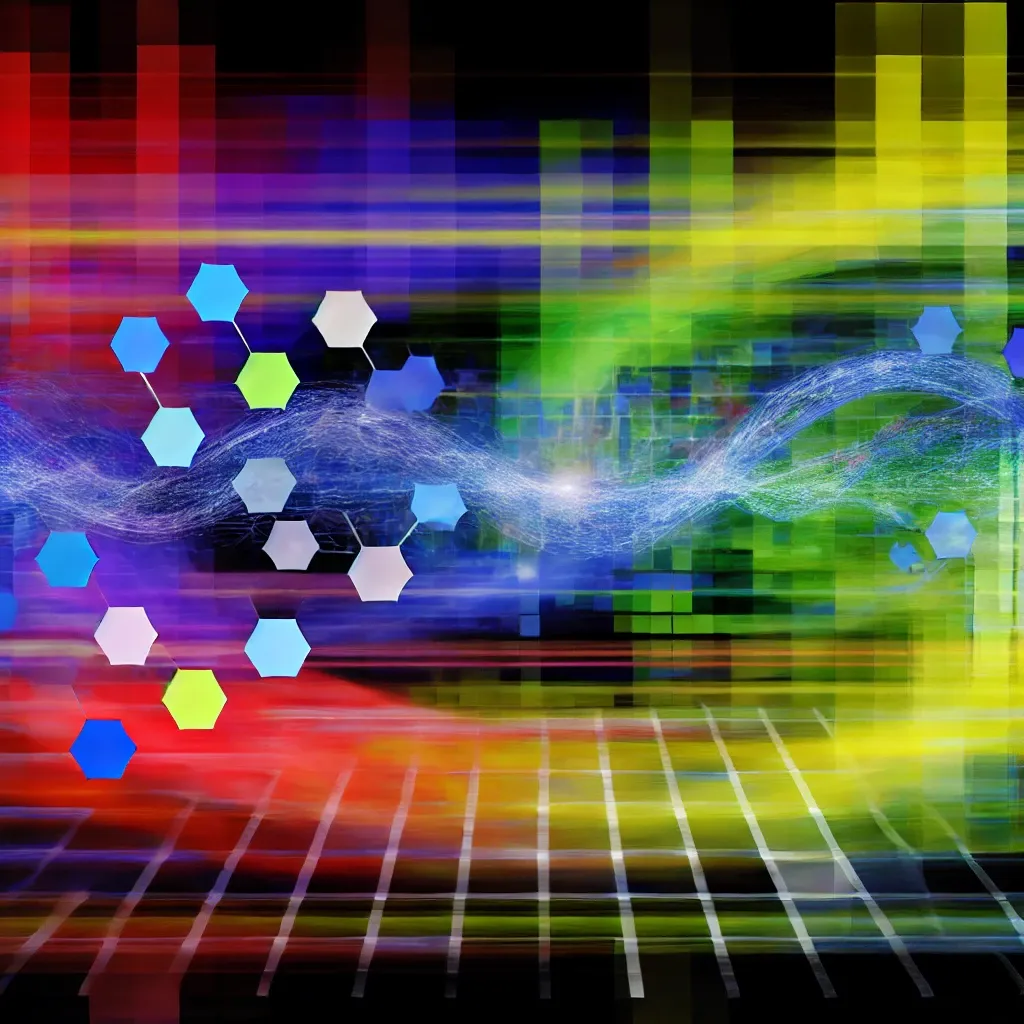
An endpoint in a REST API is a specific URL that allows clients (such as applications or services) to interact with the server to access or manipulate resources. In other words, an endpoint is the entry point or address where requests can be made to retrieve data, send information, or perform some operation related to a particular resource.
In the context of a RESTful API, resources represent entities that the server makes available to the client, such as users, products, orders, etc. Each resource has a unique address or "endpoint" that can be accessed via a URL. For example, in an API that handles user information, an endpoint might be:
https://api.example.com/users
This endpoint can be used to interact with user data. Depending on the HTTP method used (GET, POST, PUT, DELETE, etc.), different actions can be performed:
- GET /users: Retrieve the list of all users.
- POST /users: Create a new user.
- GET /users/{id}: Retrieve information about a specific user.
- PUT /users/{id}: Update information of a specific user.
- DELETE /users/{id}: Delete a specific user.
Importance of Endpoints
Endpoints are crucial for defining how clients interact with the API. Each endpoint should be clearly documented, specifying what operations can be performed, what parameters are required, and what data format is expected in both requests and responses. This makes it easier for developers to use the API correctly and ensures effective communication between client and server applications.
In summary, an endpoint is the specific "touchpoint" through which a client can interact with the resources provided by a REST API, enabling integration and communication between different applications or services.